Java is a versatile, platform-independent programming language that has been widely adopted for building applications ranging from web-based systems to mobile apps. This comprehensive Java beginner’s guide is designed for beginners looking to learn Java, covering everything from installation to core programming concepts.
Why Learn Java?
Java is one of the most popular programming languages in the world, primarily due to its:
- Portability: Write once, run anywhere (WORA) capability.
- Robustness: Strong memory management and exception handling.
- Community Support: A large ecosystem and vibrant community for assistance.
To learn more about the benefits of Java, check out Java’s Official Website.
What you’ll learn in Java Beginner’s Guide
This Java beginner’s guide will be divided into two parts:
- Part One: Contains knowledge on…
- Part Two: Which will be seen in a completely separate blog post will handle an introduction to the key concepts of Object Oriented Programming. Particularly; Classes and Objects, Inheritance, Polymorphism, and Encapsulation.
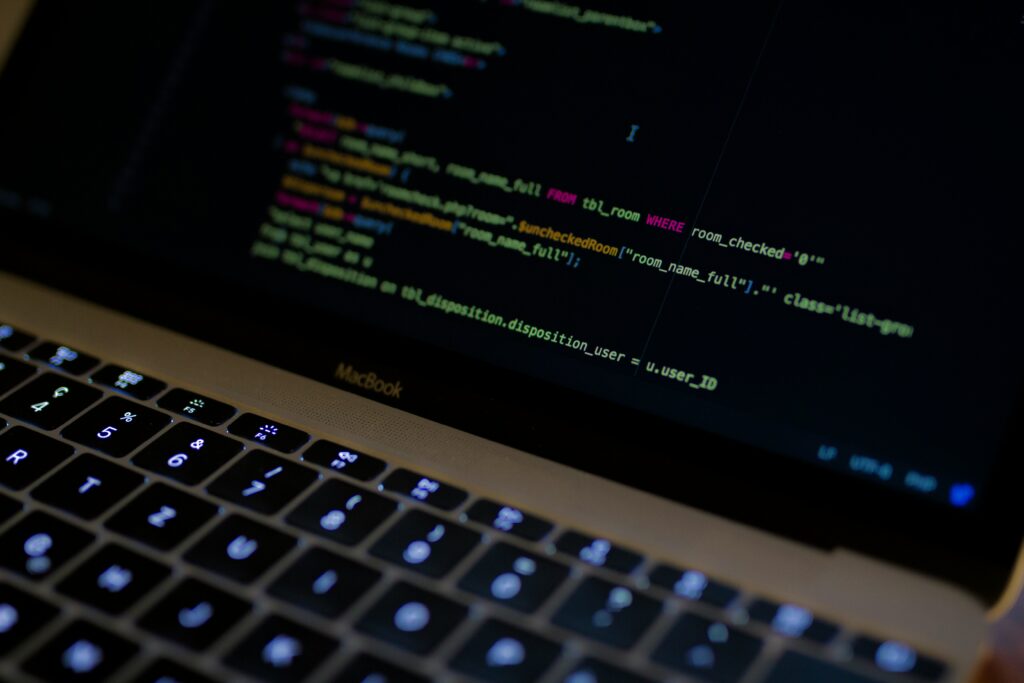
Setting Up the Java Development Environment
Before you can start coding, you’ll need to set up your Java environment:
- Download and Install the Java Development Kit (JDK):
- Visit the Oracle JDK download page and download the latest version.
- Follow the installation instructions for your operating system.
- Choose an Integrated Development Environment (IDE):
- IntelliJ IDEA: A powerful IDE with excellent features for Java development. Download IntelliJ IDEA.
- Eclipse: A popular, open-source IDE for Java. Download Eclipse.
You can get more detailed information on one of our blog articles specifically targeting Setting Up Your Java Development Environments.
Understanding Java Syntax and Data Types
Hello World Example: Java Beginner’s Guide.
Every programming journey begins with the classic “Hello, World!” example. Here’s how you can write this simple program in Java:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Data Types in Java
Java supports several data types, each serving different purposes:
- Primitive Data Types:
int
: Integer values (e.g.,int number = 10;
)double
: Floating-point numbers (e.g.,double price = 19.99;
)char
: Single characters (e.g.,char letter = 'A';
)boolean
: True or false values (e.g.,boolean isJavaFun = true;
)
- Reference Data Types: Includes arrays, classes, and interfaces.
For further reading and to gain more knowledge from our Java beginner’s guide on Java syntax and Java data types you should take a keen look at the next awesome article: Data Structures in Java.
Understanding Control Structures in Java
Java provides various control structures to manage the flow of your program. Let’s see these control structures below.
Conditional Statements
Use if
, else if
, and else
to execute code based on certain conditions:
int number = 10;
if (number > 0) {
System.out.println("Positive number");
} else {
System.out.println("Non-positive number");
}
Loops
Loops allow you to execute a block of code multiple times. Here are the two most common types:
- For Loop: Best for a known number of iterations.
for (int i = 0; i < 5; i++) { System.out.println("Iteration: " + i); }
- While Loop: Best when the number of iterations is not known in advance.
int count = 0; while (count < 5) { System.out.println("Count: " + count); count++; }
Try these out in your editor and see the results for yourself.
For in-depth examples and code challenges I recommend you visit the article: Control Structures In Java.
Conclusion to part one of our java beginner’s guide.
Congratulations! You’ve taken the first steps in learning Java programming. This guide covered setting up your environment, basic syntax & data structures, and control structures. Feel free to go ahead and take the Part Two of the Java beginner’s guide. As you continue to explore Java, consider diving into more advanced topics and projects.
Further Resources with content that may not be found in this java beginner’s guide.
- Java Documentation for official API references.
- Java Community for forums and discussions.
Happy coding!